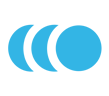
Resource Owner Flow
Getting a token to access a resource you own is simple. See below for details and examples. Remember you can find all your own client Id's and secrets in your Welcome email.
For this flow we recommend you simply create a new token for each use of the API. You can however use Refresh Tokens, but they're not covered in this Quick Start.
If you're an enterprise customer replace {enterprise_pin}/ with your enterprise PIN. This can be found in management portal page. If you're not an enterprise user, please do not include {enterprise-pin}/ before the username.
Request
POST https://identity.qflowhub.io/core/connect/tokenContent-type:
application/x-www-form-urlencoded
client_id=acme_resowner&client_secret=cb818203-d58c-4bf7-a66a-43522716cf69&username={enterprise_pin}/joe.bloggs@acme.com&password=secret1234&scope=qflowapi+openid+offline_access&grant_type=password
Response
{
"access_token" : "c6102720a52dbd822e24d567b002160c",
"expires_in" : 3600,
"token_type" : "Bearer",
"refresh_token" : "2573de01ab1b8a1d0bb3b1ac4dd0b3ca"
}
$url = 'https://identity.qflowhub.io/core/connect/token';
$clientId = 'acme_resowner';
$clientSecret = '96184159-60d4-4ad4-a11e-0ff3ddcc6678';
$username = '{enterprise_pin}/joe.bloggs@example.com';
$password = 'secret1234';
$scope = 'qflowapi+openid+offline_access';
$grantType = 'password';
$options = array(
'http' => array(
'header' => 'Content-type: application/x-www-form-urlencoded\r\n',
'method' => 'POST',
'content' => 'client_id=' .
$clientId . '&client_secret=' .
$clientSecret . '&username=' .
$username . '&password=' .
$password . '&scope=' .
$scope . '&grant_type=' .
$grantType));
$context = stream_context_create($options);
$result = file_get_contents($url, false, $context);
$obj = json_decode($result);
$accessToken = $obj->{'access_token'};
echo $accessToken;
var url = "https://identity.qflowhub.io/core/connect/token";
var clientId = "acme_resowner";
var clientSecret = "96184159-60d4-4ad4-a11e-0ff3ddcc6678";
var username = "{enterprise_pin}/joe.bloggs@example.com";
var password = "secret1234";
var scopes = "qflowapi+openid+offline_access";
var grantType = "password";
var sc = new StringContent(
"client_id=" + clientId +
"&client_secret=" + clientSecret +
"&username=" + username +
"&password=" + password +
"&scope=" + scopes +
"&grant_type=" + grantType,
UnicodeEncoding.UTF8,
"application/x-www-form-urlencoded");
using (var client = new HttpClient())
{
var result = await client.PostAsync(url, sc);
dynamic json = JsonConvert.DeserializeObject(await result.Content.ReadAsStringAsync());
var token = json.access_token;
Console.WriteLine(token.ToString());
}